How to Create a Single Page App with NextJs
17th Jan 2022
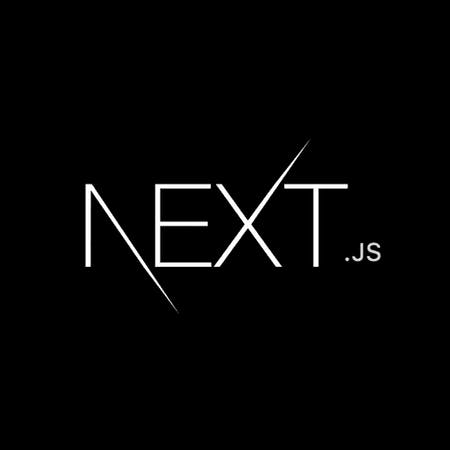
NextJs is very useful for creating static websites but how do you configure it if you are building a single page application (SPA)? The setup isn't immediately obvious.
The key thing here is we don't want to build a page on the server when the path is dynamic.
Let's take a look at how you can set it up, it's actually pretty straight-forward.
Rewrites
The first piece of this puzzle is the rewrites
function within next.config.js
.
I can add rewrites to the beforeFiles
option which will take a dynamic path and convert sections of it to query values.
In the example below I tell NextJs to render using the static page pages/contact.tsx
but I have also told NextJs to match :contactId
and make it available as a query on the router.
async rewrites() {return {beforeFiles: [{source: '/contact/:contactId',destination: '/contact',},]}}
There are a few different options for the rewrite matches, take a look at the Rewrites documentation for more information.
Page and Router
The next piece of this puzzle is to use the router to get the query value. In this example I am pulling out a :contactId
from the path.
export default function Page() {const { query } = useRouter()const contactId = query.contactIdreturn (<AuthenticatedLayout title="Contact">{contactId === 'new' ? (<AddContactForm />) : (typeof contactId === 'string' && (<ContactDetailPage contactId={contactId} />))}</AuthenticatedLayout>)}
The useRouter
hook gives me access to the query variable which is an object with key: value
. The NextJs rewrite has put the contactId on this object so we can access is directly.
We do need to do a bit of checks to make sure it is there, and that it is a basic string.
That's about it
There you go, you should notice that when you build your application the page is a simple static page. Really fast to load and it also has access to the path variable.